This article is from our Febooti archive, it was relevant then, and I think that it is still relevant today (a few details changed).
Previous article: Loops in the Batch files with examples.
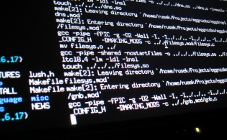
The IF command in the batch scripting is very similar to the IF statement in other programming languages. The IF command allows you to branch the execution of the batch file into two separate paths depending on some condition.
The basic syntax of IF command is:
IF {Statement} {If statement is TRUE, perform this action}
If the statement being tested is TRUE, then perform the action on the same line (to the right side of IF). If the statement is FALSE, then continue to the next line. The TRUE action statement is, essentially, ignored in case of FALSE. A GOTO command could perhaps be present on this line to send processing of the batch file to another location in the script. The IF command is great for testing three types of conditions including replaceable parameters, system ERRORLEVEL, and it can compare two strings.
Remember that several IF commands can be stacked one after another, to test more conditions, or to create tree like algorithms. An algorithm is simply your solution to the problem at hand.
Here is an example that tests replaceable parameters (Note that on some versions of Windows or a different configuration of your command prompt might change how this example is run. Play around with it and your CMD interpreter settings):
:: LOOP.BAT
:: Compares Replaceable parameters.
@ECHO OFF
:TOP
IF (%1)==() GOTO END
ECHO Value is "%1" and still running...
SHIFT
GOTO TOP
:END
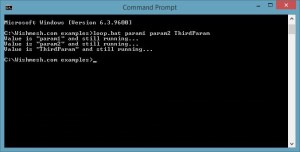
The first two lines are remark statements indicating the name of the program as well as a brief description of what the program does. The third line brings command echoing to a standstill. Line four marks off the label for sub program that a GOTO command could hop to. Line 5 tests for a blank (empty) replaceable parameter. When %1 contains any information then that information gets placed in the first set of parentheses (parameter)==() and evaluates to FALSE. This is what we want. A common mistake is to omit the parentheses, however we need them so we do not create an infinite loop.
When replaceable parameter %1 is empty, the test becomes ()==() and appropriately evaluates to TRUE. This line is the core code of this batch script. When the expression evaluates to TRUE the GOTO END command is executed. This essentially ends the program. When the expression (condition) is FALSE, the next line of code is executed. The sixth line displays the value of parameter combined in message, Value is “something” and still running… using the ECHO command. That next line of code contains the SHIFT command. We know that the SHIFT command shifts the values of replaceable parameters over. The last line is the END label symbolizing the end of the program.
Note that the indents do not change your code and are not mandatory, however it is easier to read code if we indent everything inside the IF block.
The IF command is a handy command by itself, however it yields great possibilities when combined with other commands. Such as the EXIST command. The EXIST command is used to test the existence of a file. This can be done using the following syntax scheme:
IF EXIST command syntax:
IF EXIST {filename} {If filename exist, perform this action}
Lets consider example, where we pass 3 parameters to the batch file, and assume that each of the params is a filename:
:: ExistFile.BAT
:: Example of IF EXIST command sequence.
@ECHO OFF
IF EXIST %1 ECHO File %1 exists!
IF EXIST %2 ECHO File %2 exists!
IF EXIST %3 ECHO File %3 exists!
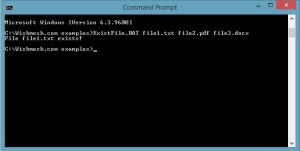
Lines 1 and 2 are remark statements indicating the name of the batch file and a brief description of what the batch file does. Line 3 turns off command echoing. Lines 4 through line 6 tests the existence of whatever values are in the replaceable parameters 1, 2, and 3 respectively. This program is rather trivial, but servers as a good example.
Read more in Microsoft help site.
Next article: MS-DOS command redirection operators.
This article is from our Febooti archive, it was relevant then, and I think that it is still relevant today (a few details changed).